Matrix Multiplication In Golang
All Implementations in this repository are written in both Python and Golang. Within the nested for loop we are multiplying every Matrix item with user given number.
If a and b both implement RawMatrixer that is they can be represented as a blas64General blas64Gemm general matrix multiplication is called while instead if b is a RawSymmetricer blas64Symm is used general-symmetric multiplication and if b is a VecDense blas64Gemv is used.
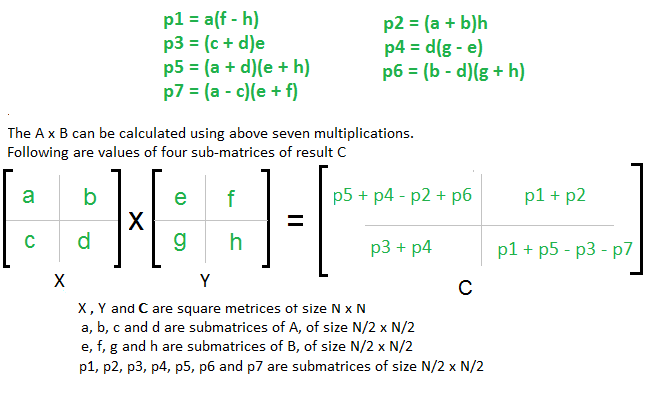
Matrix multiplication in golang. MatNewVecDense returns a matVecDense that implements a method func MulVec a matMatrix b matVector Here is a test to validate the functionality. Improve your programming skills in Go with more advanced mulithreading topics. Matmult_pimpl nil A B.
I for j 0. Understand the advantages limits and properties of Parallel computing. You cant have dynamic types.
Go Matrix Multiplication Program. Size of each matrix is 1024 x 1024. Write a Go Program to perform the Scalar Matrix Multiplication which means multiplying each matrix item with a given number.
Given below you can find algorithm and program. Take number of rows and columns as input. An array can contain only one type int OR bool not several.
Here we are going to learn algorithm and program to multiply two matrix. This isnt a matrix multiplication as pointed out above. Although be aware that Harrys patch was probably written in a hurry so he does not check for the second multiplicand to be square Strassen and Douglas only work for square matrices - same number of rows and columns.
About the method The main condition of matrix multiplication is that the number of columns of the 1st matrix must equal to the number of rows of the 2nd one. Learn about goroutines mutexes reader writers locks waitgroups channels condition variables and more. The workaround is to make an array of interface but that means youd have to make ugly type assertions to get the proper type back.
Multiply float and int numbers result is 31500000. Java Program Matrix Addition Subtraction Multiplication Transpose Posted By. Go Program to perform Scalar Matrix Multiplication.
Func TestMatrixVectorMul t testingT a matNewDense 3 3 float64 1 2 3 4 5 6 7 8 9 b matNewVecDense 3 float64 1 2 3 actual make float64 3 c matNewVecDense 3 actual this was the. The algorithms for sparse matrix is outside of that article. Single IPython Notebook contains all Algorithms given in this Part 1.
Var m3 33 int. Package main import fmt func main var i j rows columns num int var orgMat 10 10int var scalarMultiMat 10 10int fmtPrint Enter the. Else if dk Threshold.
However in Golang youll have one big problem. Apply nested for loop to take matrix elements as input. As a result of multiplication you will get a new matrix that has the same quantity of rows as the 1st one has and the.
Tutorials references and examples are constantly reviewed to avoid errors but we cannot warrant full correctness of all content. Apply multiplication on matrix elements. GO Program to Generate Multiplication Table.
The first for loop performs the matrix multiplication and assigns the values to the multiplication matrix. Here is a working code. Go matmult_pimpl sync0 A B C i0 i1 j0 mj k0 k1 matmult_pimpl nil A B C i0 i1 mj j1 k0 k1.
The second for loop prints the items in that matrix. Which is useful and gives me words to hunt for on google for better matrix-multiplication. Lets take a few input data.
There are many possible type combinations and special cases. A B C where A B C - square matrix. Develop programs with Golang that are highly Concurrent and Parallel.
In this Go matrix multiplication program we used two for loops. Package main import fmt func main var number1 int var number2 float64 number1 5 number2 63 result float64number1 number2 fmtPrintfMultiply float and int numbers result is fn result Output is. Mk k0 dk 2.
Package main import fmt func main var rows columns i j int var multimat1 10 10int var multimat2 10 10int var multiplicationnmat 10. The base of article is the performance research of matrix multiplication. May 17 2020 Matrix related programs are famous in interview which not only check the knowledge of programming but checks the basic idea of mathematics.
Here we will write a program to multiply two matrix in go. This is the implementation of 1st Part in 3-Part Series of Algorithms Illuminated Book. K m3 ij m3 ij m1 ik m2 kj twoDimensionalMatrices 333 int m1 m2 m3 matrixNames string MATRIX1 MATRIX2 MATRIX3 MATRIX1MATRIX2.
Golang Programs is designed to help beginner programmers who want to learn web development technologies or start a career in website development. J m3 ij 0. Declaring a matrix variable for holding the multiplication results.
Python golang sort recursion matrix-multiplication strassen-algorithm quick-sort closest-pair karatsuba.
Go Program To Addition Two Matrices Golang Codingster
Go Program To Find Matrix Determinant
Correct Simple Matrix Multiplication And Transpostion With Go Https Play Golang Org P Uvjlr8qaxv3 Github
Github Oelmekki Matrix Work With Matrices In Golang
Keras Convolution Neural Network Layers And Working Dataflair Network Layer Deep Learning Networking
Easy Way To Remember Strassen S Matrix Equation Geeksforgeeks
Github Konstantin8105 Matrix Multiply Part1 Optimization Of Matrix Multiplication
Github Mitsuse Matrix Go An Experimental Library For Matrix Manipulation Implemented In Golang
Go Program To Multiplication Two Matrices Golang Codingster
Why A Neural Network Without Non Linearity Is Just A Glorified Line 3d Visualization Artificial Neural Network Visualisation Networking
Go Matrix Multiplication Program
Go Program To Multiplication Two Matrices Golang Codingster
Go Program To Find Sum Of Each Matrix Row